PikaDevBoard: All-in-One STM32 Development
An all-in-one STM32F103 development board packed with sensors, display, and tons of I/O. Perfect for IoT, robotics, and embedded projects.
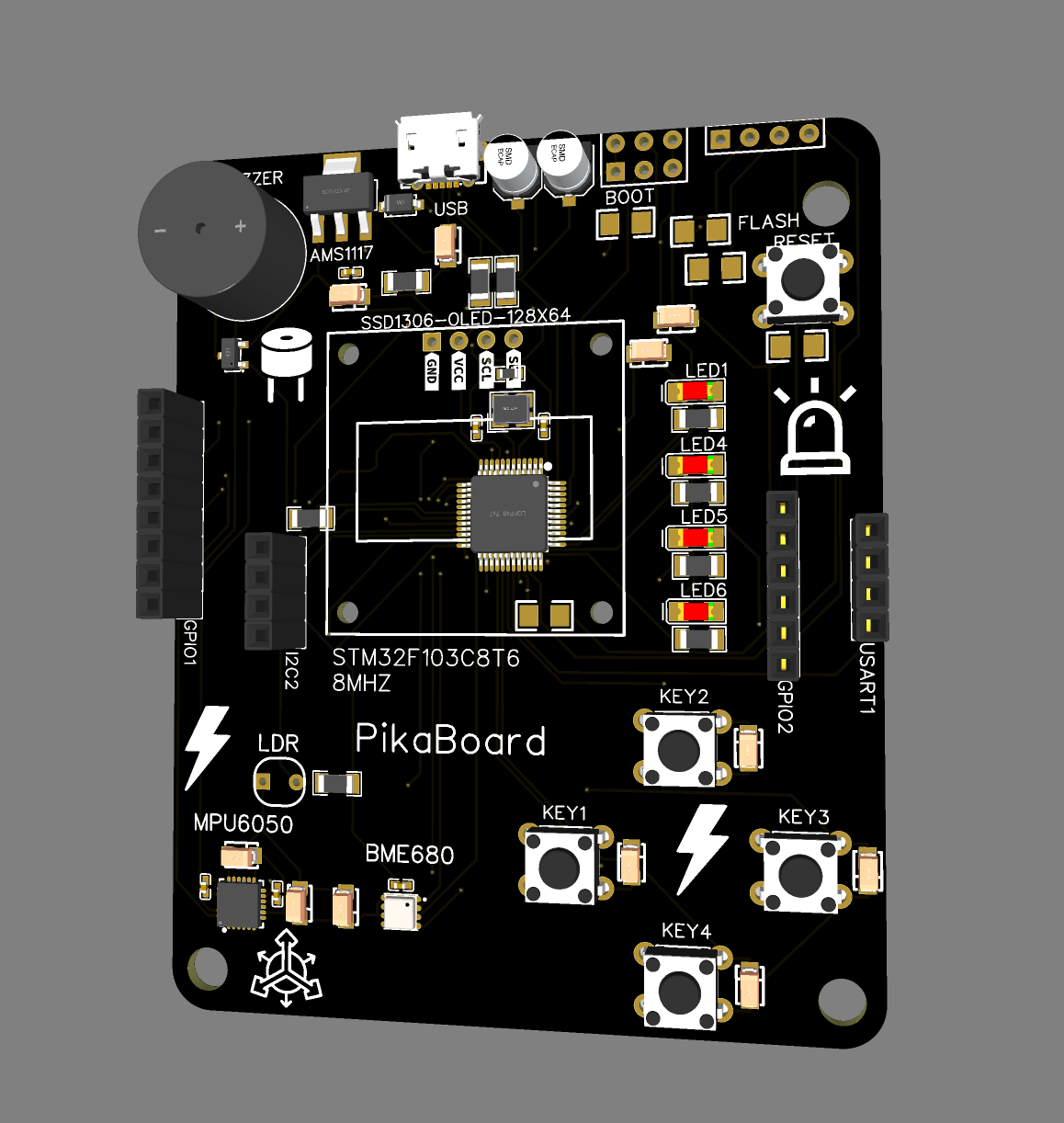
Packed with Features
STM32F103C8T6
Powerful 32-bit ARM Cortex-M3 microcontroller running at 8MHz
BME680 Sensor
Environmental sensing for temperature, humidity, pressure, and gas
MPU6050
6-axis motion tracking with accelerometer and gyroscope
OLED Display
128x64 SSD1306 OLED for visualizing data and user interfaces
Multiple I/O
Buttons, LEDs, buzzer, and expansion headers for all your project needs
Connectivity Ready
Easy integration with wireless modules and other peripherals
Technical Specifications
/* Includes ------------------------------------------------------------------*/
#include "main.h"
#include "ssd1306.h"
#include "bme680.h"
#include "mpu6050.h"
/* Private variables ---------------------------------------------------------*/
I2C_HandleTypeDef hi2c1;
SPI_HandleTypeDef hspi1;
BME680_t bme680;
MPU6050_t mpu6050;
float temperature, humidity, pressure;
int16_t accelX, accelY, accelZ;
char buffer[64];
/* Private function prototypes -----------------------------------------------*/
void SystemClock_Config(void);
static void MX_GPIO_Init(void);
static void MX_I2C1_Init(void);
int main(void)
{
/* MCU Configuration--------------------------------------------------------*/
HAL_Init();
SystemClock_Config();
/* Initialize all configured peripherals */
MX_GPIO_Init();
MX_I2C1_Init();
/* Initialize OLED display */
SSD1306_Init();
SSD1306_Clear();
SSD1306_GotoXY(0, 0);
SSD1306_Puts("PikaDevBoard", &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_GotoXY(0, 15);
SSD1306_Puts("Initializing...", &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_UpdateScreen();
/* Initialize BME680 sensor */
BME680_Init(&bme680, &hi2c1, BME680_I2C_ADDR_PRIMARY);
/* Initialize MPU6050 sensor */
MPU6050_Init(&mpu6050, &hi2c1, MPU6050_ADDR);
/* Infinite loop */
while (1)
{
/* Read BME680 sensor data */
BME680_ReadData(&bme680);
temperature = bme680.temperature;
humidity = bme680.humidity;
pressure = bme680.pressure / 100.0f; // Convert to hPa
/* Read MPU6050 accelerometer data */
MPU6050_ReadAccel(&mpu6050);
accelX = mpu6050.Accel_X_RAW;
accelY = mpu6050.Accel_Y_RAW;
accelZ = mpu6050.Accel_Z_RAW;
/* Update display */
SSD1306_Clear();
SSD1306_GotoXY(0, 0);
SSD1306_Puts("PikaDevBoard", &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_GotoXY(0, 15);
sprintf(buffer, "Temp: %.1f C", temperature);
SSD1306_Puts(buffer, &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_GotoXY(0, 25);
sprintf(buffer, "Hum: %.1f %%", humidity);
SSD1306_Puts(buffer, &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_GotoXY(0, 35);
sprintf(buffer, "Pres: %.1f hPa", pressure);
SSD1306_Puts(buffer, &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_GotoXY(0, 45);
sprintf(buffer, "Accel: %d %d %d", accelX, accelY, accelZ);
SSD1306_Puts(buffer, &Font_7x10, SSD1306_COLOR_WHITE);
SSD1306_UpdateScreen();
/* Delay */
HAL_Delay(1000);
}
}
Easy to Program
The PikaDevBoard is designed to be programmer-friendly. Use STM32CubeIDE, PlatformIO, or Arduino IDE to bring your projects to life. With comprehensive libraries and examples, you'll be up and running in minutes.
STM32 HAL Support
Full support for STM32 HAL libraries and STM32CubeIDE
Example Library
Dozens of ready-to-use code examples
Perfect For
Weather Stations
Create your own IoT weather station with the built-in BME680 environmental sensor.
- Temperature monitoring
- Humidity tracking
- Air quality sensing
Motion Projects
Build motion-controlled devices and robotics with the MPU6050 accelerometer and gyroscope.
- Gesture controls
- Robot balancing
- Motion detection
Smart Displays
Create information displays and user interfaces with the built-in OLED screen.
- Status monitors
- Smart home controls
- Data visualization
Ready to Start Building?
Get your hands on the PikaDevBoard and unlock endless possibilities for your next project.